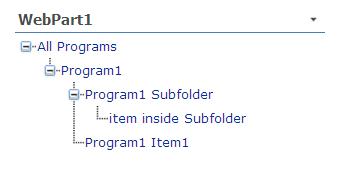
Introduction
This article provides you with the code snippet to display a SharePoint list data as a tree view WebPart.
Background
The code creates a tree view control and then populates it with various folders and subfolders from a given list. To access the subfolders of the list, an SPQuery
variable is used. The list item is first checked with a condition (listitem.Folder == null
) to determine whether it is a folder or a list item. The GetItems
recursive method is then called to get the list item or the items in the folder.
Using the code
The code displays data from a "Links
" type custom list called "All Programs" as a tree view. The CreateChildControl
method calls the GetFolder
method to access the subfolders of the list. The GetFolder
method further calls the GetItems
method to get the items or subfolders in a subfolder. GetItems
uses SPquery
to get the items in a folder and is called recursively to get the items in subfolders of a folder.
public class WebPart1 : System.Web.UI.WebControls.WebParts.WebPart
{
TreeView treeView;
TreeNode rootNode;
public WebPart1()
{
}
protected override void RenderContents(System.Web.UI.HtmlTextWriter writer)
{
base.RenderContents(writer);
}
protected override void CreateChildControls()
{
base.CreateChildControls();
SPSite currentSite = SPContext.Current.Site;
using (SPWeb currentWeb = currentSite.OpenWeb())
{
treeView = new System.Web.UI.WebControls.TreeView();
treeView.ShowLines = true;
treeView.ExpandDepth = 0;
SPList list = currentWeb.Lists["All Programs"];
rootNode = new System.Web.UI.WebControls.TreeNode(list.Title, "",
"", list.RootFolder.ServerRelativeUrl.ToString(), "");
GetFolder(list.RootFolder, rootNode, list);
treeView.Nodes.Add(rootNode);
}
this.Controls.Add(treeView);
base.CreateChildControls();
}
public void GetFolder(SPFolder folder, TreeNode rootNode, SPList list)
{
TreeNode newNode = new System.Web.UI.WebControls.TreeNode(folder.Name, "",
"~/_layouts/images/itdl.gif",
folder.ServerRelativeUrl.ToString(), "");
try
{
if (folder.Name != "Link")
{
foreach (SPFolder childFolder in folder.SubFolders)
{
if (childFolder.Name != "Link")
{
TreeNode trn =
new System.Web.UI.WebControls.TreeNode(childFolder.Name, "",
"", childFolder.ServerRelativeUrl.ToString(), "");
newNode = GetItems(childFolder, trn);
rootNode.ChildNodes.Add(newNode);
}
}
}
}
catch { }
}
public TreeNode GetItems(SPFolder folder, TreeNode node)
{
SPQuery qry = new SPQuery();
qry.Folder = folder;
SPWeb web = null;
web = folder.ParentWeb;
SPListItemCollection ic = web.Lists[folder.ParentListId].GetItems(qry);
foreach (SPListItem subitem in ic)
{
if (subitem.Folder != null)
{
TreeNode childNode =
new System.Web.UI.WebControls.TreeNode(subitem.Folder.Name);
node.ChildNodes.Add(GetItems(subitem.Folder, childNode));
}
if (subitem.Folder == null)
{
TreeNode trn1 = new System.Web.UI.WebControls.TreeNode(
subitem["Title0"].ToString());
node.ChildNodes.Add(trn1);
}
}
return node;
}
}
In the above code, SPquery
is used to get the items from a given folder since there is no other way to get items from a list of subfolders as item.folder
does not expose subfolders.
For any comments and questions on this article, please visit my blog: http://mysharepointwork.blogspot.com/2009/09/tree-view-webpart-for-list-data.html.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.